读书笔记-04-JavaScript语言精粹
大约 1 分钟
JavaScript语言精粹
简介

对象 Object
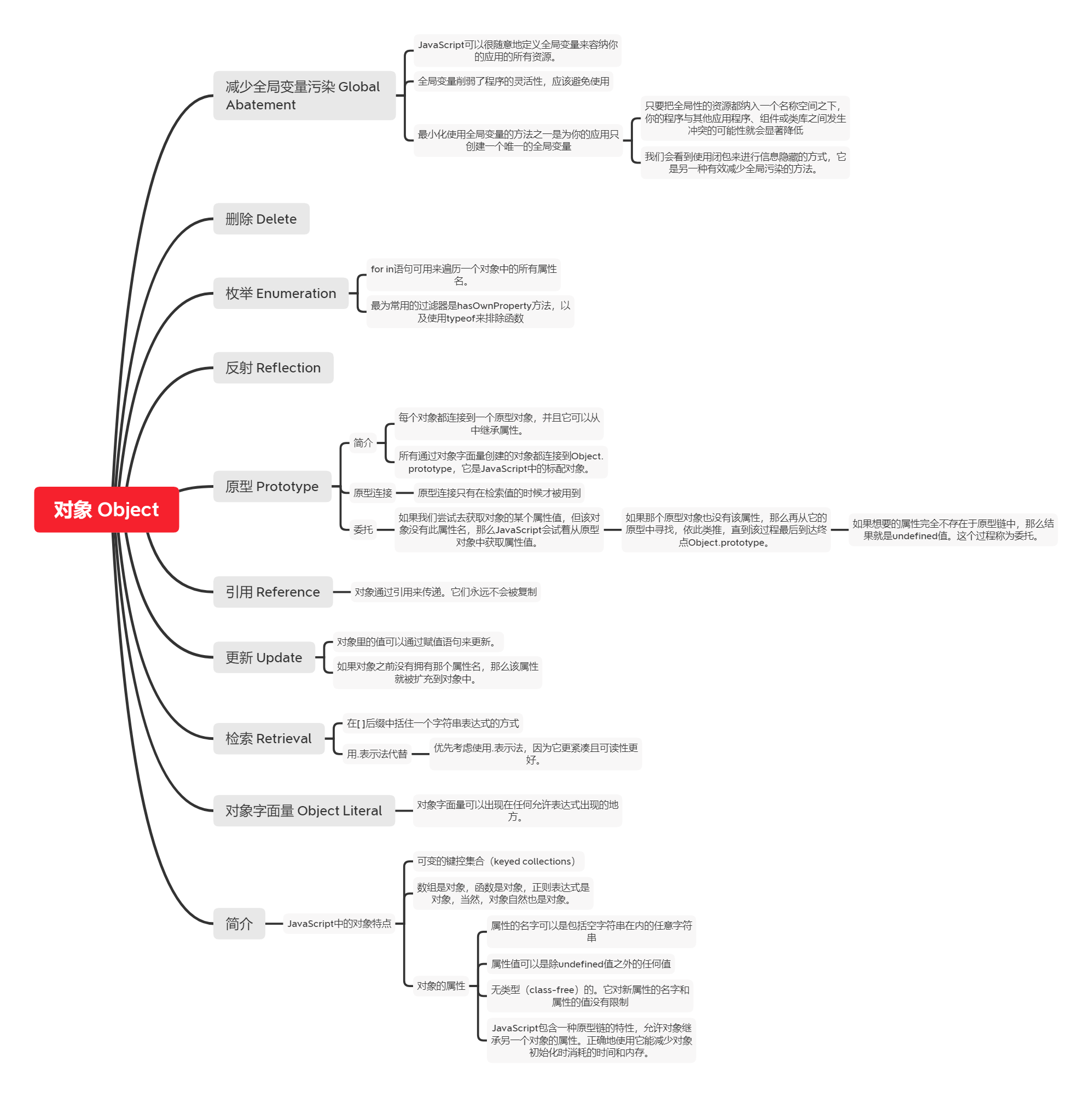
函数 Function

继承 Inheritance

数组 Array

正则表达式 Regular Expressions

方法 Methods

如何实现
Array
array.pop( )
// pop可以像这样实现:
Array.method('pop',function(){
return this.splice()(this.length - 1,1)[0]
})
array.push(item...)
// push可以像这样实现
Array.method('push',function(){
this.splice.apply(
this,
[this.length,0].concat(Array.prototype.slice.apply(arguments)))
return this.length;
})
array.shift()
Array.method('shift',function(){
return this.splice(0,1)[0];
})
array.splice(start, deleteCount, item...)
// splice可以像这样实现:
Array.method('splice',function(start,deleteCount){
var max = Math.max,
min = Math.min,
delta,
element,
insertCount = max(argments.length - 2, 0),
k = 0,
len = this.length,
new_len,
result = [],
shift_count;
start = start || 0;
if(start < 0){
start += len;
}
start = max(min(start,len),0);
deleteCount = max(min(typeof deleteCount === 'number' ? deleteCount : len,len - start),0)
delta = insertCount - deleteCount;
new_len = len + delta;
while(k < deleteCount){
element = this[start + k];
if(element !== undefined){
result[k] = element;
}
k += 1;
}
shift_count = len - start - deleteCount;
if(delta < 0){
k = start + insertCount;
while(shift_count){
this[k] = this[k-delta];
k +=1;
shift_count -= 1;
}
this.length = new_len;
}else if(delta>0){
k = 1;
while(shift_count){
this[new_len - k] = this[len - k];
k += 1;
shift_count -= 1;
}
this.length = new_len;
}
for(k = 0; k< insertCount; k+=1){
this[start += k] = arguments[k + 2];
}
return result;
})
array.unshift(item...)
// unshift可以像这样实现:
Array.methods('unshift', function(){
this.splice.apply(this,[0,0].concat(Array.prototype.slice.apply(arguments)));
return this.length;
})
RegExp
regexp.test(string)
// test可以像这样实现:
RegExp.method('test',function(string){
return this.exec(string) !== null;
})
Srting
string.charAt(pos)
// charAt可以像这样实现:
String.method('charAt',function(pos){
return this.splice(pos,pos+1);
})
代码风格 Style
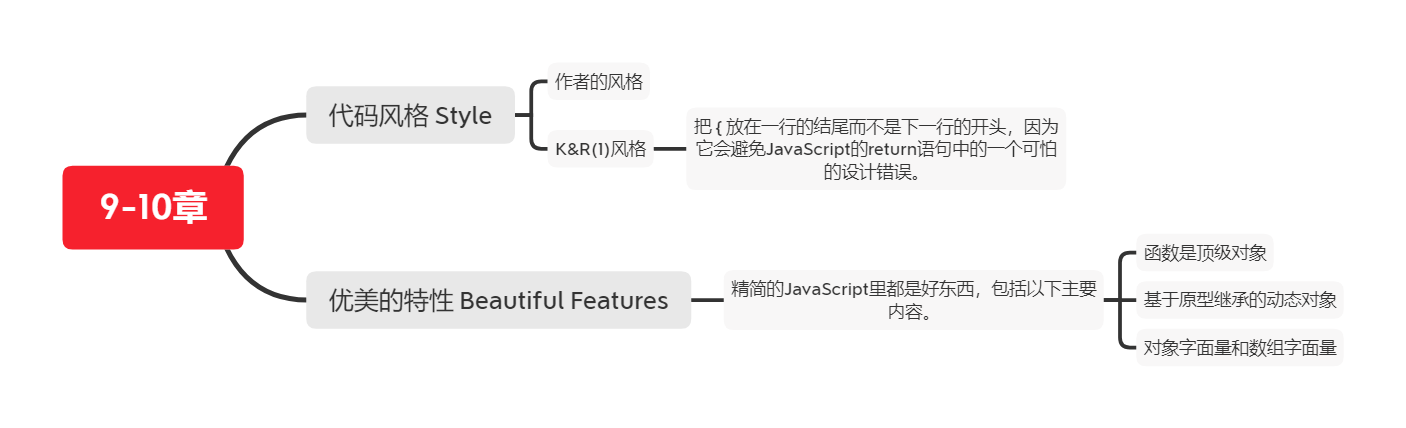
毒瘤&糟粕
